Programming in Java
Object Oriented in Java
Advanced topics in Java
Tutorials
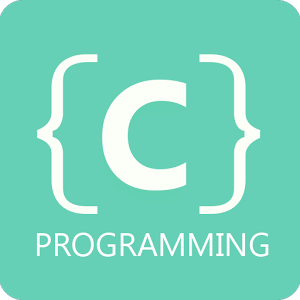
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
If-Else, Switch-Break in Java
If Else
In programming, decision making is used to specify the order in which statements are executed.
In this tutorial, you will learn to write if…else statements to make decisions in your program.
There are following types of scenarios :
1. Simple if statement
2. if – else statement
3. Nested if statement
4. if-else-if statement (if-else ladder)
General syntax of if-else is as following :
if(<condition here>)
{
// statements to be executed if the condition is true.
}
else {
// statements to be executed if the condition is false.
}
» In the above scenario, else is the optional part of if .
» When both if and else are available, one part must be executed.
Multiple if :
You can use multiple if statements to apply multiple conditions.
if(<condition1>)
{
}
if(<condition2>)
{
}
if(<condition3>)
{
}
» In this scenario, all if conditions are independent of each other so, any of them may execute .
» All the above three sections may be executed if all the three conditions are true.
» If all the conditions are false, then none of the above will be executed.
Nested if :
Another If may be there within an if. In this case, inner section executes only if both outer and inner sections are true.
if(<condition 1>)
{
// statement 1
if(<condition 2>)
{
//statement2
}
//statement3
}
» In this scenario statement 1 & statement 3 execute if condition 1 is true,
» but statement 2 executes only if both condition 1 & condition 2 are true.
IF else ladder :
if else ladder may be explained as following example.
if(<condition 1>)
{
// statement 1
}
else
{
if(<condition 2>)
{
// statement 2
}
else
{
// statement 3
}
}
» In this case, the if else ladder is in such a manner that either statement 1 or statement 2 or statement 3 executes.
Switch In Programming :
If we have the scenario of nested if, then switch statement is faster than nested if…else (but not always).
Also, the syntax of switch statement is cleaner and easy to understand.
In a switch statement, we provide a value that is to be compared with a value associated with each option.
Whenever the given value matches with the value associated with an option, the execution starts from that option. In switch statement every option is defined as a case.
Break Statement :
The break statement in programming is used for two following purposes :
break can be used to terminate a case in the switch statement as in above given example.
break statement inside a loop is used to terminate the program control, and resumes at the next statement following the loop.
Break statement breaks the loop even if the condition is true ( explained in next section with loop examples).