Programming in Java
Object Oriented in Java
Advanced topics in Java
Modal Box
Tutorials
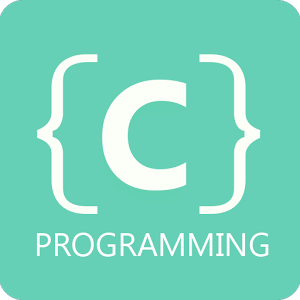
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
AWT and Event
AWT stands for Abstract Window Toolkit. AWT controls are heavyweight and platform dependent.
AWT controls are such as Button , Label, TextField etc and java.awt package, need to be imported for this.
AWT provides GUI like an applet but it is run by main method, not like the applet.
GUI windows can be created by any of the container class, like Frame or Panel etc.
Here, we are creating a GUI window by using Frame and few AWT controls
import java.awt.*; class FrameDemo { FrameDemo()//constructor { Frame f=new Frame(); f.setLayout(new Flowlayout());// Many layouts are available so we can use as per required view. Button b=new Button("OK"); Label l=new Label("This is Label");
f.add(l); f.add(b); f.setSize(300,300); } public static void main(String args[]) { FrameDemo f=new FrameDemo(); } }
⇒ Here when we click on Button, its clicked, but no working is there with the click of the button.
To better understand the functioning of button click and event, the following program shows sum or substraction of two numbers
import java.awt.*; import java.awt.event.*; class FrameDemo implements ActionListener { FrameDemo() //constructor { Frame f=new Frame(); f.setLayout(new Flowlayout());// Many layouts are available so we can use // as per required view. Label l= new Label("Add/Substraction Program"); Label l1= new Label("Number1"); Label l2= new Label("Number1"); Label l3= new Label("result"); TextField t1= new TextField(10); TextField t2= new TextField(10); TextField t3= new TextField(10); Button b1=new Button("Sum"); Button b2=new Button("Diff"); f.add(l); f.add(l1); f.add(t1); f.add(l2); f.add(t2); f.add(l3); f.add(t3); f.add(b1); f.add(b2); b1.addActionListener(this); b2.addActionListener(this); f.setSize(600,400); }
public void actionPerformed(ActionEvent ae)
{
String s=ae.getActionCommand();
int n1=Integer.parseInt(t1.getText());
int n2=Integer.parseInt(t1.getText());
int result=0;
if(s.equals("Sum"))
result=n1+n2;
if(s.equals("Diff"))
result=n1-n2;
t3.setText(""+result);
}
public static void main(String args[])
{
FrameDemo f=new FrameDemo();
}
}
Video/ C Introduction
Watch video in full size