Programming in Java
Object Oriented in Java
Advanced topics in Java
Modal Box
Tutorials
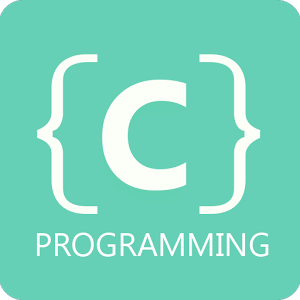
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Multithreading in java
With the help of multithreading, multiple threads may run simultaneously.
A thread is used to achieve multitasking and it is a light weight component.
main method is itself a thread in java.
class MainThreadDemo
{
public static void main(String args[])
{
Thread t=Thread.currentThread();
System.out.println("Thread details:"+t);
//output main,5,main
t.setName("myThread");
System.out.println("Name of thread"+t.getName());
System.out.println("Priority of thread"+t.getPriority());
}
}
Thread class may be created either by extending Thread class or implementing Runnable interface.
class NewThread implements Runnable {
Thread t;
NewThread() {
// Create a new, second thread
t = new Thread(this, "Demo Thread");
System.out.println("Child thread: " + t);
t.start(); // Start the thread
}
// This is the entry point for the second thread.
public void run() {
try {
for(int i = 5; i > 0; i--) {
System.out.println("Child Thread: " + i);
Thread.sleep(500);
}
} catch (InterruptedException e) {
System.out.println("Child interrupted.");
}
System.out.println("Exiting child thread.");
}
}
class ThreadDemo {
public static void main(String args[]) {
new NewThread(); // create a new thread
try {
for(int i = 5; i > 0; i--) {
System.out.println("Main Thread: " + i);
Thread.sleep(1000);
}
} catch (InterruptedException e) {
System.out.println("Main thread interrupted.");
}
System.out.println("Main thread exiting.");
}
}
Ball moving game using Thread and applet.
import java.awt.*;
import java.applet.*
/*<applet code="game" widht=400 height=500>
</applet>
*/
public class game extends Applet implements Runnable
{
Thread t;
int i=10;
public void init()
{
t= new Thread(this);
t.start();
}
public void run()
{
for(; ; )//infinite loop
{
try
{
t.sleep(50)
i=i+2;
if(i>=400) //to check the ball goes outside the applet window.
i=10;
repaint();
}
catch(Exception ex)
{
}
}
}
public void paint(Graphics g)
{
g.drawOval(i,20,40,40);
}
}
Video/ C Introduction
Watch video in full size