Introduction
C Programming
C Advanced
Important C Programs
If - Else:
In programming, decision making is used to specify the order in which statements are executed. In this tutorial, you will learn to write if…else statements to make decisions in your program.
The if statement verifies the given condition and decides whether a block of statements are executed or not based on the condition’s result. In C, the if statement is classified into four types as follows …
- Simple if statement
- if – else statement
- Multiple if statement
- Nested if statement
- if-else-if statement (if-else ladder)
An if statement can be followed by an optional else statement, which executes when the Boolean expression is false.
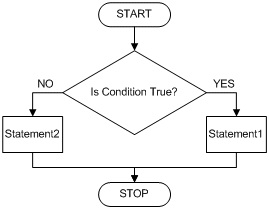
1 Simple if statement
//Syntax for if statement
if(condition)
{
/*statement to be executed for true condition*/
}
#include<stdio.h>
#include<conio.h> int main() { int n=0; printf("enter the number to check\n"); scanf("%d",&n); if(n%2==0) {
printf("%d is even",n);
} }
Input: 10 Output: 10 is even
Input 15 Output: No output as else is not there(as we know else is an optional part.)
2 if - else
if(condition)
{
/*statement to be executed for true condition*/
}
else
{
/*statement to be executed for false condition */
}
Program to check if given no. is Even or Odd using if else
#include<stdio.h>
#include<conio.h> int main() { int n=0; printf("enter the number to check\n"); scanf("%d",&n); if(n%2==0) {
printf("%d is even",n);
}
else
{
printf("%d is odd",n);
}
}
Input: 10 Output: 10 is even (if part executes because if condition is true)
Input 15 15 is odd (else part executes because if condition is not true)
3 Multiple If :
if(condition)
{
/*statement to be executed for this condition*/
}
if(condition)
{
/*statement to be executed for this condition */
}
Program to find check even or odd number using only if
any number of if may be there based on the conditions.
In this scenario all if are independent on each other .
which if part will execute depends on the its condition no matters with other if.
#include<stdio.h>
#include<conio.h> int main() { int n=0; printf("enter the number to check\n"); scanf("%d",&n); if(n%2==0) {
printf("%d is even",n);
}
if(n%2!=0)
{
printf("%d is odd",n);
}
}
Input: 10 Output: 10 is even (first if part executes because first if condition is true).
Input 15 15 is odd (second if part executes because second if condition is true).
4 Nested If :
if(condition)
{
if(condition)
{
/*statement to be executed if both if are true */
}
}
#include<stdio.h>
#include<conio.h> int main() { int n=0; printf("enter the number to check between 0 to 50\n"); scanf("%d",&n); if(n>0) {
if(n<50)
{
printf("%d is between 0 and 50 ",n);
} // end of inner if
} // end of outer if
}
Input: 20 Output: 20 is between 0 to 50(as both if condition is true).
Input -10 No output (first outer if condition is false so it will not even check inner if).
In this case inner if executes only if outer if also true. So if i enter a number between
0 to 50 it will execute because for that both conditions are true.
5 If Else ladder:
if(condition)
{
}
else
{
if(condition)
{
/*statement to be executed if both if are true */
}
else
{
}
} //end of outer else
// Program to check the number is zero even or odd
#include<stdio.h>
#include<conio.h> int main() { int n=0; printf("enter the number to check "); scanf("%d",&n); if(n==0) {
printf("number is zero");
}
else
{
if(n%2==0)
{
printf("%d is even number",n);
}
else
{
printf("%d is odd number",n);
}
}
}
Input: 0 Output: number is zero
Input -10 No is even
Input-15 No is odd
This if else ladder may go upto any level.