Introduction to python
Collection in Python
Object Oriented python
Python With MYSQL And Excel
Python GUI
Programs in Python
- Swap two number
- Calculate the area
- Even Odd or Zero
- Largest ,Middle and Smallest Number
- Calculate Telephone Bill
- Print Table of The given Number
- Factorial of the number
- Reverse and check number is palindrome
- check number is prime , armstrong
- Program to Print the given patterns
- Guess A Number Game using Random
Click Here
Tutorials
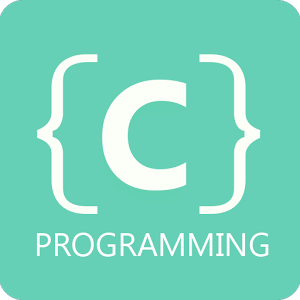
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Using Python and Tkinter we can create database driven GUI applications as following
Student Entry Tkinter
Using Python Tkinter GUI registration page may be created and record may be inserted in database making the database connection.
import tkinter
from tkinter import Entry
import mysql.connector
from tkinter import messagebox
global roll
global name
def ok():
try:
db = mysql.connector.connect(
host="localhost",
user="root",
passwd="",
database="sharp_db")
mycursor = db.cursor()
sql = "insert into student(rollno,name) values (%s,%s)"
messagebox.showinfo("Status", "msg1")
roll="4095"
name="john"
messagebox.showinfo("Status", "msg2")
val=(roll,name)
mycursor.execute(sql, val)
db.commit()
print("inserted")
messagebox.showinfo("Status", "Student Registered")
except:
messagebox.showinfo("Error", "Exception occured")
window = tkinter.Tk()
window.title("Student Registration Page")
tkinter.Label(window, text = "Roll No : ",bg="red",fg="yellow").grid(row=0,column=0)
username=Entry(window).grid(row = 0, column = 4,padx=25)
tkinter.Label(window, text = "Name ",bg="green",fg ="yellow").grid(row=0,column=8,padx=10)
password=tkinter.Entry(window).grid(row=0,column=9,padx=25,pady=25)
button3=tkinter.Button(window, text = "Click",bg="red",fg="yellow",command=ok).grid(row=0,column=12)
window.mainloop()
when we run the above code output is:
Click Here
Tutorials
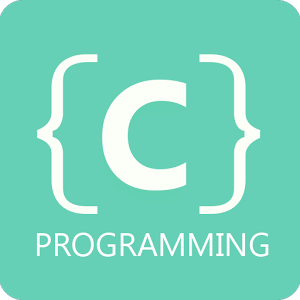
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Video/ C Introduction
Watch video in full size