Introduction
C Programming
C Advanced
Important C Programs
Modal Box
Tutorials
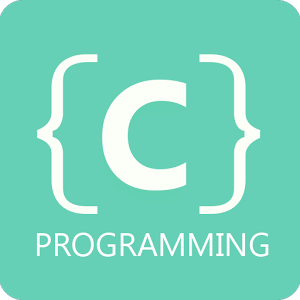
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Stack
Stack implements LIFO (Last In First Out) or Stack is a linear data structure in which the insertion and deletion operations are performed at only one end.
The following operations are performed on the stack …
1. Push (To insert an element on to the stack)
2. Pop (To delete an element from the stack)
3. Display (To display elements of the stack)
Stack data structure can be implemented either using Array or using Linked List.
Program to implement Stack using Array.
#include<stdio.h> #include<conio.h>
#define MAX 10
int top=-1, stack[MAX]; void push(); int pop(); void display();
void main() { int ch, ele=0; char choice='y'; do { printf("\n** Stack Menu **"); printf("\n\n1.Push\n2.Pop\n3.Display\n4.Exit"); printf("\n\nEnter your choice"); scanf("%d", &ch);
switch(ch) { case 1: printf("enter the element to be inserted"); scanf("%d", &ele); push(ele);
break;
case 2: ele=pop(); printf("poped item is %d", ele); break;
case 3: display(); break; case 4: exit(0);
default: printf("\nWrong Choice!!"); } printf("Do you want to continue(y/n) \n"); scanf(" %c", &choice);
} while(choice=='y'); }
void push(int ele) { if(top==MAX-1) { printf("\nStack is full!!"); } else { stack[++top]=ele; } }
int pop() { int ele=0; if(top==-1) { printf("\nStack is empty!!"); } else { ele=stack[top--]; } return ele; }
void display() { int i; if(top==-1) { printf("\nStack is empty!!"); } else { printf("\nStack elements are...\n"); for(i=top; i>=0; i--) printf("%d\n", stack[i]); } }
Video/ C Introduction
Watch video in full size