Introduction
C Programming
C Advanced
Important C Programs
Modal Box
Tutorials
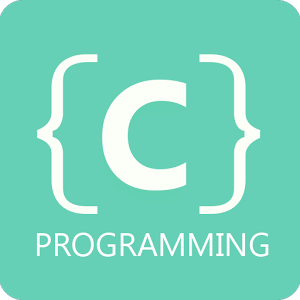
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Pointer
Pointer is a special type of variable used to store the memory location address of a variable.
A pointer variable may be declared as following:
int *ptr;
it can store the address but not the actual value.
Example to implement the working of pointers
#include <stdio.h> #include <conio.h> void main() { int i = 3, *ptr ; ptr = &i ; // pointer variable is storing address. //two ways to display the address printf("Address of i =%d ", &i) ; printf("Address of i =%d ", ptr) ; printf("Value of variable i = %d ", *ptr) ; //value can be used by using ptr (pointer variable). printf("value of variable i = %d ", i) ; }
Call By Value :
When we pass the normal value to a function, as we did in function topic, then it is known as call by value.
#include <stdio.h> #include <conio.h> void main() { int n=0, f=0; printf("enter the number to check factorial"); scanf("%d",&n); f=fact(n); printf("factorial of %d=%d ",n,f); } int fact(int n) { int f=1; while(n>0) { f=f*n; n--; } return f; }
Call By Reference :
If instead of passing the actual value, only the reference is passed, then it is known as call by reference.
#include<stdio.h>
#include<conio.h>
void main() { int a=10, b=20; printf("a= %d, b=%d", a,b) ; // a=10, b=20 change(&a,&b); printf("a= %d, b=%d", a,b) ; // a=20, b=40 changed value }
void change(int *a, int *b)
{
*a=(*a)*2;.//change value here
*b=(*b)*2;
}
Video/ C Introduction
Watch video in full size