Basic Programs
If Else based Programs
Loop Based Programs
Array Based Programs
String Programs
Function Programs
Pointers Programs
Structure & File Handling Programs
Data Structure Programs
Modal Box
Tutorials
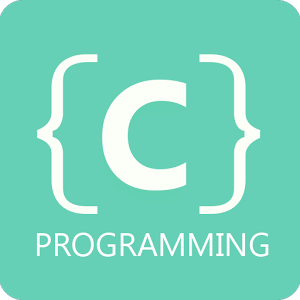
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
String based programs
Find length of string and count vowel in string
#include<stdio.h> #include<conio.h>
void main() {
int i=0,count=0;
char str[80];
printf("enter the string");
gets(str);
while(str[i]!=NULL)
{
if(str[i]=='a'||str[i]=='e'||str[i]=='i'||str[i]=='o'||str[i]=='u')
{
count++;
}
i++;
}
printf("string length=%d no of vowels=%d",i,count);
}
C Program to reverse string and check if it is palindrome
#include<stdio.h> #include<conio.h>
void main() {
int i=0,lastIndex=0,count=0,flag=0;
char str[80],str1[80];
printf("enter the string");
gets(str);
while(str[i]!=NULL)
{
i++;
}
lastIndex=i-1;
i=0;
while(str[i]!=NULL)
{
str1[i]=str[lastIndex];
if(str[i]!=str1[i])
{
flag=1;
}
i++;
lastIndex--;
}
str1[i]=NULL;
printf("reverse string is\n");
puts(str1);
if(flag==0)
printf("the given string is palindrome");
else
printf("not palindrome");
}
C program to check substring in the given string
#include<conio.h>
#include<stdio.h>
void main()
{
int i=0,j=0;
char str[50],substr[50];
clrscr();
printf("Enter the string : ");
gets(str);
printf("Enter substring : ");
gets(substr);
while(str[i]!=NULL && substr[j]!=NULL)
{
if(str[i]!=substr[j])
{
i++;
j=0;
}
else
{
j++;
i++;
}
}
if(substr[j]==NULL)
printf("substring Found");
else
printf("Not found");
}
C Program to replace a word with another in string
#include<stdio.h> #include<conio.h>
void main() {
int i=0,count=0;
char str[80];
printf("enter the string\n");
gets(str);
while(str[i]!=NULL)
{
if(str[i]=='a'&& str[i+1]=='n' && str[i+2]=='d')//replace and with the
{
str[i]='t;
str[i+1]='h';
str[i+2]='e';
i=i+2;
}
i++;
}
printf("the change string is ");
puts(str);
}
C Program to count four letter word in given string
#include<conio.h>
#include<stdio.h>
void main()
{
int i=0,j=0, k=0;
char d[50], c[50];
clrscr();
printf("Enter the string : ");
gets(d);
for(i=0;d[i]!=NULL;i++)
{
j=0;
while(d[i]!=32 && d[i]!=NULL)
{
j++;
i++;
}
if(d[i]==NULL)
i--;
if(j==4)
{
k=k+1;
}
}
printf("Four letter word are : %d", k);
}
Video/ C Introduction
Watch video in full size