Introduction
C Programming
C Advanced
Important C Programs
Tutorials
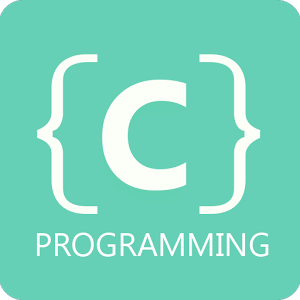
C is a very powerful and widely used language. It is used in many scientific programming situations. It forms (or is the basis for) the core of the modern languages Java and C++. It allows you access to the bare bones of your computer.C++ is used for operating systems, games, embedded software, autonomous cars and medical technology, as well as many other applications. Don't forget to visit this section...
Functions in C Language
Every C program must contain atleast one function called main(). However a program may also contain other functions.
In programming, large program may be divided into the basic building blocks known as function. The function contains the set of programming statements enclosed by {}. A function can be called multiple times to provide reusability and modularity to the C program. A program may have one or more functions.
Function's important aspects :
1. Function Declaration (Function Prototype) :-
A function must be declared globally in a c program to tell the compiler about the function name, function parameters, and return type.
2. Function Definition :-
This block contains the statements to be executed. Any function may return only single value and return statement is the last statement in the function.
If function is returning the value we have to declare the return type of the function .If function does not return any value we give void return type.
3. Function calling :-
A Function can be called anywhere in the program. The parameter to be passed to a function and argument in calling function must also be the same order and same no of arguements.
In c langauge there are many inbuilt functions. User defined functions may also be declared as following:
<return-type> <functionname> () { //statements to be executed }
Program to calculate the factorial of a given number with the help of function.
#include <stdio.h> #include <conio.h> void main() { int n=0, f=0; printf("enter the number to check factorial"); scanf("%d",&n); f=fact(n); printf("factorial of %d=%d ",n,f); } int fact(int n) { int f=1; while(n>0) { f=f*n; n--; } return f; }